Create a file-selector dialog
int PtFileSelection( PtWidget_t *parent,
PhPoint_t const *pos,
char const *title,
char const *root_dir,
char const *file_spec,
char const *btn1,
char const *btn2,
char const *format,
PtFileSelectionInfo_t *info,
int flags );
ph
This function
creates a file-selector dialog that lets the user browse files
and directories. The dialog allows the selection of a file and/or
directory and fills a PtFileSelectionInfo_t structure with
information about the selected item and the dialog.
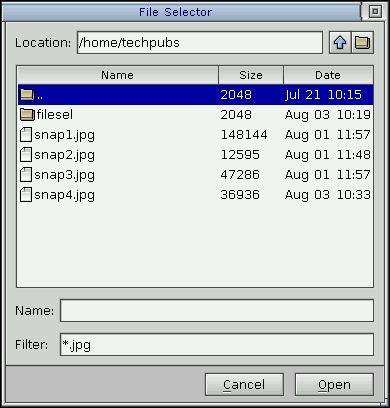
An example of the dialog created by PtFileSelection().
 |
Be sure to initialize the PtFileSelectionInfo_t structure
pointed to by info before calling this function.
This structure includes some pointers that must be set to NULL
if you don't want to provide callback functions.
For more information, see
"PtFileSelectionInfo_t structure,"
below. |
You can specify the dimensions of the dialog by setting the
info->dim field before calling this function.
This function can select directories as well as files.
Enable directory selection with the Pt_FSR_SELECT_DIRS
flag.
Existing directories can be selected with btn1 (the Open button).
PtFileSelection() can create and delete directories and delete
files.
You can create new directories at any time by pressing the New button.
When the PtFileSel widget has focus, two new hotkeys
are activated: the Insert key creates a new directory
just like the New Directory button, and the Delete key
removes the currently selected item.
This function has its own event-processing loop.
The arguments are as follows:
- parent
- The dialog's parent, which can be NULL.
It's used with the pos argument to position the dialog.
- pos
- A pointer to a
PhPoint_t
structure that's used with the parent to position the dialog.
If pos is NULL, the dialog is centered on the
screen; if parent
is NULL, the dialog is placed at the absolute coordinates of
pos; otherwise it's placed at the relative offset of
pos within parent.
- title
- The dialog's title. If NULL, the string
File Selector is used.
- root_dir
- The current directory for the file selector widget. The default is
/ if this parameter is NULL.
You can pass
a directory or a full path for a file.
PtFileSelection() parses the string and uses the longest
existing path as its root directory.
The rest of the string is used as a suggested path that's displayed
in the Name field.
- file_spec
- The file specification to look for. The default is * if
this parameter is NULL.
- btn1
- The string for button 1. The default is Open.
This is the button that returns the selected-file
information. When activated, it sets info->ret
to Pt_FSDIALOG_BTN1.
If you want to have a hotkey for this button, place an ampersand (&)
in front of the appropriate character in the string. For example, to
have the string Select with s as a hotkey,
pass &Select as btn1.
- btn2
- The string for button 2. The default is Cancel.
When activated, it sets info->ret to
Pt_FSDIALOG_BTN2.
If you want to have a hotkey for this button, place an ampersand (&)
in front of the appropriate character in the string.
- format
- A string to be used with the
Pt_ARG_FS_FORMAT
resource
of the PtFileSel widget.
It determines what information is displayed and in what order. If you
don't want the divider shown, pass NULL as this parameter,
and only the filenames are be displayed. See the description of
PtFileSel for the
format of this string.
- info
- This is a mandatory parameter. The function returns the selected file
in the path portion of this structure.
For more information, see
"PtFileSelectionInfo_t structure,"
below.
- flags
- Flags that affect the appearance and behavior of the
dialog.
The default is to show all information, and
corresponds to a value of 0. The possible values are:
- Pt_FSR_NO_FCHECK
- Permit non-existent files/directories to be selected.
(The path must exist.)
- Pt_FSR_NO_FSPEC
- Don't display the file specification.
- Pt_FSR_NO_UP_BUTTON
- Don't display the up-directory button.
- Pt_FSR_NO_NEW
- Disable new directory creation via the Insert key.
- Pt_FSR_NO_NEW_BUTTON
- Don't display the new-directory button.
- Pt_FSR_NO_SELECT_FILES
- Files aren't selectable.
- Pt_FSR_SELECT_DIRS
- Directories are selectable.
- Pt_FSR_CREATE_PATH
- Create directories as needed when typed in manually.
- Pt_FSR_NO_CONFIRM_CREATE_PATH
- Don't confirm directory creation.
- Pt_FSR_NO_DELETE
- Disable deletions via the Delete key.
- Pt_FSR_NO_CONFIRM_DELETE
- Don't confirm deletions.
- Pt_FSR_RECURSIVE_DELETE
- Enable the recursive deletion of directories.
- Pt_FSR_CONFIRM_EXISTING
- Confirm the selection of an existing file with an message.
The PtFileSelectionInfo_t structure includes
at least the following members:
- short ret
- The return code; either
Pt_FSDIALOG_BTN1 or Pt_FSDIALOG_BTN2.
- char path[PATH_MAX + NAME_MAX + 1]
- The full path of the selected item.
- PhDim_t dim
- A
PhDim_t
structure that defines the dimensions of the dialog when the selection was
completed.
You can specify the size of the dialog by setting this field before calling
PtFileSelection().
- PhPoint_t pos
- The position of the dialog when the selection was completed.
- char format[80]
- The format string of the dialog when the selection was completed.
- char fspec[80]
- The file specification of the dialog when the selection was completed.
- void *user_data
- User data to pass as the data argument
to the confirm_display,
confirm_selection, and new_directory functions.
- int (*confirm_display)
( PtWidget_t *widget,
void *data,
PtCallbackInfo_t *cbinfo)
- A function that's called before an item is added to the file selector.
If this member isn't NULL, it must point to a function.
The members of the
PtCallbackInfo_t
structure are used as follows:
This function should return Pt_CONTINUE
or Pt_END to indicate whether or not the item
should be displayed in the file selector.
- int (*confirm_selection)
( PtWidget_t *widget,
void *data,
PtCallbackInfo_t *cbinfo)
- A function that's called when the user has made a final selection
of a directory item by double-clicking on an item in the
file selector, pressing Enter in the Name box, or
clicking on the Open button.
If this member isn't NULL, it must point to a function.
The members of the
PtCallbackInfo_t
structure are used as follows:
If confirm_selection returns Pt_CONTINUE,
PtFileSelection() exits.
If confirm_selection returns Pt_END,
PtFileSelection() doesn't exit, the
selector stays on the screen, and the user must choose another
file or directory.
Applications can use this function to screen selections and avoid
having to call PtFileSelection() repeatedly.
- int (*new_directory)
( PtWidget_t *widget,
void *data,
PtCallbackInfo_t *cbinfo)
- A function that's called whenever PtFileSelection()
creates a new directory.
If this member isn't NULL, it must point to a function.
The members of the
PtCallbackInfo_t
structure are used as follows:
- reason -- Pt_CB_FSR_DIRECTORY
- reason_subtype -- one of:
- Pt_CB_FSR_DIR_AUTO
- The directory was created automatically.
- Pt_CB_FSR_DIR_MANUAL
- The directory was created by an explicit command.
- cbdata -- a pointer to a
PtFileSelectorInfo_t structure
(see below).
The function should return Pt_CONTINUE.
- PtArg_t *args
- An array of
PtArg_t
structures that store resource settings.
- int num_args
- The number of entries in the args array.
The args and num_args entries of this structure can be
used to set the following flags for the
PtFileSel
created by this function:
- Pt_FS_CASE_INSENSITIVE
- The file name's filtering (according to the file_spec ) is
case-insensitive.
- Pt_FS_FREE_ON_COLLAPSE
-
Free items on every collapse.
This means that every time an item expands, all its child items are reread
from the disk.
- Pt_FS_NO_ROOT_DISPLAY
-
Don't show the root item.
- Pt_FS_SEEK_KEY
-
Keyboard seek mode, valid only for single-level mode.
Type characters to seek an item.
- Pt_FS_SHOW_DIRS
-
Show directories.
- Pt_FS_SHOW_ERRORS
-
Show files that had a read error.
- Pt_FS_SHOW_FILES
-
Show files.
- Pt_FS_SHOW_HIDDEN
-
Show hidden files or
directories. This flag must be combined with
Pt_FS_SHOW_FILES and/or Pt_FS_SHOW_DIRS.
A hidden file or directory is one whose name begins with a period
(.).
- Pt_FS_SINGLE_LEVEL
-
Single-level mode, instead
of tree mode. Directories and possibly files are shown in one level
with a .. item for moving up directory levels.
For example:
PtFileSelectionInfo_t info;
PtArg_t args[10];
PtSetArg( &args[0], Pt_ARG_FS_FLAGS, xxxx, xxxx );
info.args = &args;
info.num_args = 1;
PtFileSelection(......, info, ....);
You can also specify the following resources in the the resource array :
The PtFileSelectorInfo_t structure is passed as
a parameter to the confirm_display, confirm_selection
and new_directory functions.
It contains at least the following members:
- char *path
- The full path of the directory item.
- struct stat *statbuf
- A pointer to the same buffer as lstatbuf
if lstat() succeeded and the file isn't a symbolic link.
If the file is a symbolic link according to lstat()
(or, perhaps, if lstat() failed),
stat()
is called, and statbuf points to its results -- or is
NULL if stat() fails.
- struct stat *lstatbuf
- A pointer to the stat structure returned by
lstat(),
or NULL if lstat() failed.
- void *user_data
- A pointer to arbitrary data to be passed to the confirmation functions.
- 0
- Success.
- -1
- An error occurred.
This callback displays a file-section dialog that lists the JPEG
files in a given directory:
/* Standard headers */
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
/* Toolkit headers */
#include <Ph.h>
#include <Pt.h>
#include <Ap.h>
/* Local headers */
#include "abimport.h"
#include "proto.h"
int
print_it( PtWidget_t *widget, ApInfo_t *apinfo,
PtCallbackInfo_t *cbinfo )
{
PtFileSelectionInfo_t info;
/* eliminate 'unreferenced' warnings */
widget = widget, apinfo = apinfo, cbinfo = cbinfo;
// Be sure to initialize the PtFileSelectionInfo_t
// structure!
memset (&info, 0, sizeof(PtFileSelectionInfo_t));
PtFileSelection( widget, NULL, "File Selector",
"/home/techpubs", "*.jpg", NULL,
NULL, NULL, &info, 0);
return( Pt_CONTINUE );
}
Photon
Safety: | |
Interrupt handler |
No |
Signal handler |
No |
Thread |
No |
PhDim_t,
PtArg_t
PtFileSel
in the Photon Widget Reference
"Dialog modules"
in the Working with Modules chapter of the
Photon Programmer's Guide